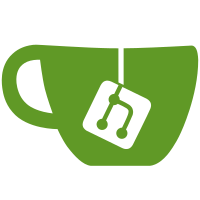
This implements an asynchronous message system using a long-poll request to the server. The server provides an endpoint /receive_messages which blocks until either a timeout occurs or we receive a batch of asynchronous messages. We send this request asynchronously and poll it 4 times a second to see if we have received any messages. The messages may either be simply for display (such as startup progress) or diagnostics, which override the diagnostics returned by OnFileReqdyToParse. In the former case, we simply display the message, accepting that this might be overwritten by any other message (indeed, requiring this), and for the latter we fan out diagnostics to any open buffer for the file in question. Unfortunately, Vim has bugs related to timers when there is something displayed (such as a "confirm" prompt or other), so we suspend background timers when doing subcommands to avoid vim bugs. NOTE: This requires a new version of Vim (detected by the presence of the particular functions used).
114 lines
3.8 KiB
Python
114 lines
3.8 KiB
Python
# Copyright (C) 2017 YouCompleteMe contributors
|
|
#
|
|
# This file is part of YouCompleteMe.
|
|
#
|
|
# YouCompleteMe is free software: you can redistribute it and/or modify
|
|
# it under the terms of the GNU General Public License as published by
|
|
# the Free Software Foundation, either version 3 of the License, or
|
|
# (at your option) any later version.
|
|
#
|
|
# YouCompleteMe is distributed in the hope that it will be useful,
|
|
# but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
# MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
# GNU General Public License for more details.
|
|
#
|
|
# You should have received a copy of the GNU General Public License
|
|
# along with YouCompleteMe. If not, see <http://www.gnu.org/licenses/>.
|
|
from __future__ import unicode_literals
|
|
from __future__ import print_function
|
|
from __future__ import division
|
|
from __future__ import absolute_import
|
|
# Not installing aliases from python-future; it's unreliable and slow.
|
|
from builtins import * # noqa
|
|
|
|
import mock
|
|
import requests
|
|
|
|
|
|
class FakeResponse( object ):
|
|
"""A fake version of a requests response object, just about suitable for
|
|
mocking a server response. Not usually used directly. See
|
|
MockServerResponse* methods"""
|
|
def __init__( self, response, exception ):
|
|
self._json = response
|
|
self._exception = exception
|
|
self.status_code = requests.codes.ok
|
|
self.text = not exception
|
|
|
|
def json( self ):
|
|
if self._exception:
|
|
return None
|
|
return self._json
|
|
|
|
|
|
def raise_for_status( self ):
|
|
if self._exception:
|
|
raise self._exception
|
|
|
|
|
|
class FakeFuture( object ):
|
|
"""A fake version of a future response object, just about suitable for
|
|
mocking a server response as generated by PostDataToHandlerAsync.
|
|
Not usually used directly. See MockAsyncServerResponse* methods"""
|
|
def __init__( self, done, response = None, exception = None ):
|
|
self._done = done
|
|
|
|
if not done:
|
|
self._result = None
|
|
else:
|
|
self._result = FakeResponse( response, exception )
|
|
|
|
|
|
def done( self ):
|
|
return self._done
|
|
|
|
|
|
def result( self ):
|
|
return self._result
|
|
|
|
|
|
def MockAsyncServerResponseDone( response ):
|
|
"""Return a fake future object that is complete with the supplied response
|
|
message. Suitable for mocking a response future within a client request. For
|
|
example:
|
|
|
|
server_message = {
|
|
'message': 'this message came from the server'
|
|
}
|
|
|
|
with patch.object( ycm._message_poll_request,
|
|
'_response_future',
|
|
new = MockAsyncServerResponseDone( [] ) ) as mock_future:
|
|
ycm.OnPeriodicTick() # Uses ycm._message_poll_request ...
|
|
"""
|
|
return mock.MagicMock( wraps = FakeFuture( True, response ) )
|
|
|
|
|
|
def MockAsyncServerResponseInProgress():
|
|
"""Return a fake future object that is incomplete. Suitable for mocking a
|
|
response future within a client request. For example:
|
|
|
|
with patch.object( ycm._message_poll_request,
|
|
'_response_future',
|
|
new = MockAsyncServerResponseInProgress() ):
|
|
ycm.OnPeriodicTick() # Uses ycm._message_poll_request ...
|
|
"""
|
|
return mock.MagicMock( wraps = FakeFuture( False ) )
|
|
|
|
|
|
def MockAsyncServerResponseException( exception ):
|
|
"""Return a fake future object that is complete, but raises an exception.
|
|
Suitable for mocking a response future within a client request. For example:
|
|
|
|
exception = RuntimeError( 'Check client handles exception' )
|
|
with patch.object( ycm._message_poll_request,
|
|
'_response_future',
|
|
new = MockAsyncServerResponseException( exception ) ):
|
|
ycm.OnPeriodicTick() # Uses ycm._message_poll_request ...
|
|
"""
|
|
return mock.MagicMock( wraps = FakeFuture( True, None, exception ) )
|
|
|
|
|
|
# TODO: In future, implement MockServerResponse and MockServerResponseException
|
|
# for synchronous cases when such test cases are needed.
|