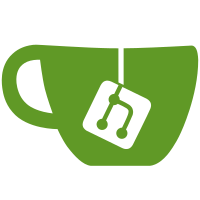
We now use the compilation working directory for a file that is specified in the CompilationDatabase. We don't actually change the working directory of the process, even temporarily (that would be annoying to users); we munge the flags coming from the database so that all the relative paths in them are resolved to absolute ones.
119 lines
3.3 KiB
Python
119 lines
3.3 KiB
Python
import os
|
|
import ycm_core
|
|
from clang_helpers import PrepareClangFlags
|
|
|
|
# Set this to the absolute path to the folder containing the
|
|
# compilation_database.json file to use that instead of 'flags'. See here for
|
|
# more details: http://clang.llvm.org/docs/JSONCompilationDatabase.html
|
|
compilation_database_folder = ''
|
|
|
|
# These are the compilation flags that will be used in case there's no
|
|
# compilation database set.
|
|
flags = [
|
|
'-Wall',
|
|
'-Wextra',
|
|
'-Werror',
|
|
'-Wc++98-compat',
|
|
'-Wno-long-long',
|
|
'-Wno-variadic-macros',
|
|
'-fexceptions',
|
|
'-DNDEBUG',
|
|
# THIS IS IMPORTANT! Without a "-std=<something>" flag, clang won't know which
|
|
# language to use when compiling headers. So it will guess. Badly. So C++
|
|
# headers will be compiled as C headers. You don't want that so ALWAYS specify
|
|
# a "-std=<something>"
|
|
'-std=c++11',
|
|
# ...and the same thing goes for the magic -x option which specifies the
|
|
# language that the files to be compiled are written in. This is mostly
|
|
# relevant for c++ headers.
|
|
'-x',
|
|
'c++',
|
|
'-isystem',
|
|
'../BoostParts',
|
|
'-isystem',
|
|
# This path will only work on OS X, but extra paths that don't exist are not
|
|
# harmful
|
|
'/System/Library/Frameworks/Python.framework/Headers',
|
|
'-isystem',
|
|
'../llvm/include',
|
|
'-isystem',
|
|
'../llvm/tools/clang/include',
|
|
'-I',
|
|
'.',
|
|
'-isystem',
|
|
'./tests/gmock/gtest',
|
|
'-isystem',
|
|
'./tests/gmock/gtest/include',
|
|
'-isystem',
|
|
'./tests/gmock',
|
|
'-isystem',
|
|
'./tests/gmock/include'
|
|
]
|
|
|
|
if compilation_database_folder:
|
|
database = ycm_core.CompilationDatabase( compilation_database_folder )
|
|
else:
|
|
database = None
|
|
|
|
|
|
def DirectoryOfThisScript():
|
|
return os.path.dirname( os.path.abspath( __file__ ) )
|
|
|
|
|
|
def MakeRelativePathsInFlagsAbsolute( flags, working_directory ):
|
|
if not working_directory:
|
|
return flags
|
|
new_flags = []
|
|
make_next_absolute = False
|
|
path_flags = [ '-isystem', '-I', '-iquote', '--sysroot=' ]
|
|
for flag in flags:
|
|
to_append = flag
|
|
|
|
if make_next_absolute:
|
|
make_next_absolute = False
|
|
if not flag.startswith( '/' ):
|
|
to_append = os.path.join( working_directory, flag )
|
|
|
|
for path_flag in path_flags:
|
|
if flag == path_flag:
|
|
make_next_absolute = True
|
|
break
|
|
|
|
if flag.startswith( path_flag ):
|
|
path = flag[ len( path_flag ): ]
|
|
to_append = path_flag + os.path.join( working_directory, path )
|
|
break
|
|
|
|
if to_append:
|
|
new_flags.append( to_append )
|
|
return new_flags
|
|
|
|
|
|
def FlagsForFile( filename ):
|
|
if database:
|
|
# Bear in mind that database.FlagsForFile does NOT return a python list, but
|
|
# a "list-like" StringVec object
|
|
working_directory = database.CompileCommandWorkingDirectoryForFile(
|
|
filename )
|
|
raw_flags = database.FlagsForFile( filename )
|
|
final_flags = PrepareClangFlags(
|
|
MakeRelativePathsInFlagsAbsolute( raw_flags,
|
|
working_directory ),
|
|
filename )
|
|
|
|
# NOTE: This is just for YouCompleteMe; it's highly likely that your project
|
|
# does NOT need to remove the stdlib flag. DO NOT USE THIS IN YOUR
|
|
# ycm_clang_options IF YOU'RE NOT 100% YOU NEED IT.
|
|
try:
|
|
final_flags.remove( '-stdlib=libc++' )
|
|
except ValueError:
|
|
pass
|
|
else:
|
|
relative_to = DirectoryOfThisScript()
|
|
final_flags = MakeRelativePathsInFlagsAbsolute( flags, relative_to )
|
|
|
|
return {
|
|
'flags': final_flags,
|
|
'do_cache': True
|
|
}
|