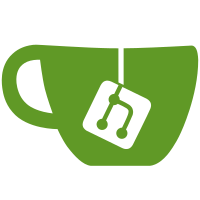
We're now able to: - Block tty updates while in URL selection mode -> no fuckups in ncurses clients anymore, hooray! - Select multiline URLs as such - Scroll nicely
272 lines
5.4 KiB
Perl
272 lines
5.4 KiB
Perl
#! perl -w
|
|
# Author: Bert Muennich
|
|
# Website: http://www.github.com/muennich/urxvt-perls
|
|
# Based on: http://www.jukie.net/~bart/blog/urxvt-url-yank
|
|
# License: GPLv2
|
|
|
|
# Use keyboard shortcuts to select URLs.
|
|
# This should be used as a replacement for the default matcher extension,
|
|
# it also makes URLs clickable with the middle mouse button.
|
|
|
|
# Usage: put the following lines in your .Xdefaults:
|
|
# - URxvt.perl-ext-common: url-select
|
|
# - URxvt.keysym.M-u: perl:url-select:select_next
|
|
|
|
# Use Meta-u to activate URL selection mode, then use the following keys:
|
|
# - 'n': select next URL (also with Meta-u)
|
|
# - 'N': select previous URL
|
|
# - Return: open selected URL in browser and quit selection mode
|
|
# - 'y': copy (yank) selected URL and quit selection mode
|
|
# - Escape: cancel URL selection mode
|
|
|
|
# Options:
|
|
# - URxvt.urlLauncher: browser/command to open selected URL with
|
|
# - URxvt.underlineURLs: if set to true, all URLs get underlined
|
|
|
|
|
|
use strict;
|
|
|
|
my $url_matcher = qr{(
|
|
(?:https?://|ftp://|news://|mailto:|file://|www\.)
|
|
[ab-zA-Z0-9\-\@;\/?:&=%\$_.+!*\x27(),~#]+[ab-zA-Z0-9\-\@;\/?&=%\$_+!*\x27()~]
|
|
)}x;
|
|
|
|
|
|
sub on_start {
|
|
my ($self) = @_;
|
|
|
|
eval { require Regexp::Common::URI };
|
|
if(!$@) {
|
|
require Regexp::Common;
|
|
Regexp::Common->import('URI');
|
|
$url_matcher = $Regexp::Common::RE{URI}{HTTP};
|
|
}
|
|
|
|
# read resource settings
|
|
$self->{browser} = $self->x_resource('urlLauncher') || 'x-www-browser';
|
|
if ($self->x_resource('underlineURLs') eq 'true') {
|
|
$self->{underline} = 1;
|
|
}
|
|
|
|
()
|
|
}
|
|
|
|
|
|
sub on_line_update {
|
|
my ($self, $row) = @_;
|
|
|
|
if ($self->{underline}) {
|
|
my $line = $self->line($row);
|
|
my $text = $line->t;
|
|
my $rend = $line->r;
|
|
|
|
while ($text =~ /$url_matcher/g) {
|
|
my $url = $1;
|
|
my ($beg, $end) = ($-[1], $+[1] - 1);
|
|
--$end if $url =~ /["')]$/;
|
|
|
|
for (@{$rend}[$beg .. $end]) {
|
|
$_ |= urxvt::RS_Uline;
|
|
}
|
|
$line->r($rend);
|
|
}
|
|
}
|
|
|
|
()
|
|
}
|
|
|
|
|
|
sub on_user_command {
|
|
my ($self, $cmd) = @_;
|
|
|
|
if ($cmd eq 'url-select:select_next') {
|
|
if (not $self->{active}) {
|
|
activate($self);
|
|
}
|
|
select_next($self, -1);
|
|
}
|
|
|
|
()
|
|
}
|
|
|
|
|
|
sub key_press {
|
|
return 1;
|
|
}
|
|
|
|
|
|
sub key_release {
|
|
my ($self, $event, $keysym) = @_;
|
|
my $char = chr($keysym);
|
|
|
|
if ($keysym == 0xff1b) {
|
|
# escape
|
|
deactivate($self);
|
|
return 1;
|
|
} elsif ($keysym == 0xff0d) {
|
|
# return
|
|
$self->exec_async($self->{browser}, ${$self->{found}[$self->{n}]}[4]);
|
|
deactivate($self);
|
|
return 1;
|
|
} elsif ($char eq 'y') {
|
|
$self->selection(${$self->{found}[$self->{n}]}[4]);
|
|
$self->selection_grab($event->{time});
|
|
deactivate($self);
|
|
return 1;
|
|
} elsif ($char eq 'n') {
|
|
select_next($self, -1);
|
|
return 1;
|
|
} elsif ($char eq 'N') {
|
|
select_next($self, 1);
|
|
return 1;
|
|
}
|
|
|
|
()
|
|
}
|
|
|
|
|
|
sub on_button_release {
|
|
my ($self, $event) = @_;
|
|
|
|
if ($self->{active}) {
|
|
if ($event->{button} == 4 || $event->{button} == 5) {
|
|
return;
|
|
} else {
|
|
return 1;
|
|
}
|
|
}
|
|
|
|
my $mask = $self->ModLevel3Mask | $self->ModMetaMask |
|
|
urxvt::ShiftMask | urxvt::ControlMask;
|
|
|
|
if ($event->{button} == 2 && ($event->{state} & $mask) == 0) {
|
|
my $col = $event->{col};
|
|
my $line = $self->line($event->{row});
|
|
my $text = $line->t;
|
|
|
|
while ($text =~ /$url_matcher/g) {
|
|
my ($url, $beg, $end) = ($1, $-[0], $+[0]);
|
|
|
|
if ($url =~ s/["')]$//) {
|
|
--$end;
|
|
}
|
|
if ($col >= $beg && $col <= $end) {
|
|
$self->exec_async($self->{browser}, $url);
|
|
return 1;
|
|
}
|
|
}
|
|
}
|
|
|
|
()
|
|
}
|
|
|
|
|
|
sub select_next {
|
|
# $dir < 0: up, > 0: down
|
|
my ($self, $dir) = @_;
|
|
my $row = $self->{row};
|
|
|
|
if (($dir < 0 && $self->{n} > 0) ||
|
|
($dir > 0 && $self->{n} < $#{ $self->{found} })) {
|
|
# another url on current line
|
|
$self->{n} += $dir;
|
|
hilight($self);
|
|
return;
|
|
}
|
|
|
|
while (($dir < 0 && $row > $self->top_row) ||
|
|
($dir > 0 && $row < $self->nrow - 1)) {
|
|
my $line = $self->line($row);
|
|
$row = ($dir < 0 ? $line->beg : $line->end) + $dir;
|
|
$line = $self->line($row);
|
|
my $text = $line->t;
|
|
|
|
if ($text =~ /$url_matcher/g) {
|
|
delete $self->{found};
|
|
|
|
do {
|
|
my ($beg, $end) = ($-[0], $+[0]);
|
|
--$end if $& =~ /['")]$/;
|
|
push @{$self->{found}}, [$line->coord_of($beg),
|
|
$line->coord_of($end), substr($text, $beg, $end - $beg)];
|
|
} while ($text =~ /$url_matcher/g);
|
|
|
|
$self->{row} = $row;
|
|
$self->{n} = $dir < 0 ? $#{$self->{found}} : 0;
|
|
hilight($self);
|
|
return;
|
|
}
|
|
}
|
|
|
|
deactivate($self) unless $self->{found};
|
|
|
|
()
|
|
}
|
|
|
|
|
|
sub hilight {
|
|
my ($self) = @_;
|
|
|
|
if ($self->{found}) {
|
|
if ($self->{row} < $self->view_start() ||
|
|
$self->{row} >= $self->view_start() + $self->nrow) {
|
|
# scroll selected url into visible area
|
|
my $top = $self->{row} - ($self->nrow >> 1);
|
|
$self->view_start($top < 0 ? $top : 0);
|
|
}
|
|
|
|
$self->want_refresh();
|
|
}
|
|
|
|
()
|
|
}
|
|
|
|
|
|
sub refresh {
|
|
my ($self) = @_;
|
|
|
|
if ($self->{found}) {
|
|
$self->scr_xor_span(@{$self->{found}[$self->{n}]}[0 .. 3], urxvt::RS_RVid);
|
|
}
|
|
|
|
()
|
|
}
|
|
|
|
sub activate {
|
|
my ($self) = @_;
|
|
|
|
$self->{active} = 1;
|
|
|
|
$self->{row} = $self->view_start() + $self->nrow;
|
|
$self->{n} = 0;
|
|
$self->{view_start} = $self->view_start();
|
|
$self->{pty_ev_events} = $self->pty_ev_events(urxvt::EV_NONE);
|
|
|
|
$self->enable(
|
|
key_press => \&key_press,
|
|
key_release => \&key_release,
|
|
refresh_begin => \&refresh,
|
|
refresh_end => \&refresh,
|
|
);
|
|
|
|
()
|
|
}
|
|
|
|
|
|
sub deactivate {
|
|
my ($self) = @_;
|
|
|
|
$self->disable("key_press", "key_release", "refresh_begin", "refresh_end");
|
|
$self->view_start($self->{view_start});
|
|
$self->pty_ev_events($self->{pty_ev_events});
|
|
|
|
if ($self->{found}) {
|
|
delete $self->{found};
|
|
$self->want_refresh();
|
|
}
|
|
|
|
$self->{active} = 0;
|
|
|
|
()
|
|
}
|