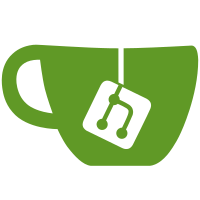
This fixes the bug which caused floating windows to be visible even when switching to a different workspace. Instead of ignoring a specific sequence, we now set an ignore_unmap counter for each container. (So, should containers be closed too early or stay open even if they should be closed, we probably need to have a closer look at the counter. At the moment, it is increased by one on reparenting and unmapping (for workspace changes) and decremented by one on each UnmapNotify event). This system is better because a sequence does not describe a single unmap or reparent request but a request to X11 on the network layer -- which can contain multiple requests.
65 lines
1.6 KiB
Perl
65 lines
1.6 KiB
Perl
#!perl
|
|
# vim:ts=4:sw=4:expandtab
|
|
# Regression test: Floating windows were not correctly unmapped when switching
|
|
# to a different workspace.
|
|
|
|
use i3test tests => 5;
|
|
use X11::XCB qw(:all);
|
|
use Time::HiRes qw(sleep);
|
|
|
|
BEGIN {
|
|
use_ok('X11::XCB::Window');
|
|
}
|
|
|
|
my $i3 = i3("/tmp/nestedcons");
|
|
|
|
my $tmp = get_unused_workspace();
|
|
$i3->command("workspace $tmp")->recv;
|
|
|
|
#############################################################################
|
|
# 1: open a floating window, get it mapped
|
|
#############################################################################
|
|
|
|
my $x = X11::XCB::Connection->new;
|
|
|
|
# FIXME: we open a tiling container as long as t/37* is not done (should swap positions with t/36* then)
|
|
my $twindow = $x->root->create_child(
|
|
class => WINDOW_CLASS_INPUT_OUTPUT,
|
|
rect => [ 0, 0, 30, 30],
|
|
background_color => '#C0C0C0',
|
|
);
|
|
|
|
isa_ok($twindow, 'X11::XCB::Window');
|
|
|
|
$twindow->map;
|
|
|
|
sleep 0.25;
|
|
|
|
# Create a floating window which is smaller than the minimum enforced size of i3
|
|
my $window = $x->root->create_child(
|
|
class => WINDOW_CLASS_INPUT_OUTPUT,
|
|
rect => [ 0, 0, 30, 30],
|
|
background_color => '#C0C0C0',
|
|
# replace the type with 'utility' as soon as the coercion works again in X11::XCB
|
|
window_type => $x->atom(name => '_NET_WM_WINDOW_TYPE_UTILITY'),
|
|
);
|
|
|
|
isa_ok($window, 'X11::XCB::Window');
|
|
|
|
$window->map;
|
|
|
|
sleep 0.25;
|
|
|
|
ok($window->mapped, 'Window is mapped');
|
|
|
|
# switch to a different workspace, see if the window is still mapped?
|
|
|
|
my $otmp = get_unused_workspace();
|
|
$i3->command("workspace $otmp")->recv;
|
|
|
|
sleep 0.25;
|
|
|
|
ok(!$window->mapped, 'Window is not mapped after switching ws');
|
|
|
|
$i3->command("nop testcase done")->recv;
|