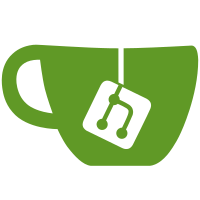
Before commit 4976fa3350
, setting the
layout of workspaces to something else than the default would just mess
up the parent container of the workspace (the content container).
After that commit, it would create an unnecessary split container when
you change the layout _before_ opening any containers. To avoid this, we
now store the layout (similar to how the 'workspace_layout'
configuration directive works) and apply it when the first container is
attached to the workspace.
Fixes #796
119 lines
2.8 KiB
Perl
119 lines
2.8 KiB
Perl
#!perl
|
|
# vim:ts=4:sw=4:expandtab
|
|
#
|
|
# Please read the following documents before working on tests:
|
|
# • http://build.i3wm.org/docs/testsuite.html
|
|
# (or docs/testsuite)
|
|
#
|
|
# • http://build.i3wm.org/docs/lib-i3test.html
|
|
# (alternatively: perldoc ./testcases/lib/i3test.pm)
|
|
#
|
|
# • http://build.i3wm.org/docs/ipc.html
|
|
# (or docs/ipc)
|
|
#
|
|
# • http://onyxneon.com/books/modern_perl/modern_perl_a4.pdf
|
|
# (unless you are already familiar with Perl)
|
|
|
|
use i3test;
|
|
use List::Util qw(first);
|
|
|
|
# to not depend on List::MoreUtils
|
|
sub all (&@) {
|
|
my $cb = shift;
|
|
for (@_) {
|
|
return 0 unless $cb->();
|
|
}
|
|
return 1;
|
|
}
|
|
|
|
sub none (&@) {
|
|
my $cb = shift;
|
|
for (@_) {
|
|
return 0 if $cb->();
|
|
}
|
|
return 1;
|
|
}
|
|
|
|
my $i3 = i3(get_socket_path());
|
|
|
|
####################
|
|
# Request tree
|
|
####################
|
|
|
|
my $tree = $i3->get_tree->recv;
|
|
|
|
# a unique value
|
|
my $ignore = \"";
|
|
|
|
my $expected = {
|
|
fullscreen_mode => 0,
|
|
nodes => $ignore,
|
|
window => undef,
|
|
name => 'root',
|
|
orientation => $ignore,
|
|
type => 0,
|
|
split => JSON::XS::false,
|
|
id => $ignore,
|
|
rect => $ignore,
|
|
window_rect => $ignore,
|
|
geometry => $ignore,
|
|
swallows => $ignore,
|
|
percent => undef,
|
|
layout => 'splith',
|
|
floating => 'auto_off',
|
|
last_split_layout => 'splith',
|
|
scratchpad_state => 'none',
|
|
focus => $ignore,
|
|
focused => JSON::XS::false,
|
|
urgent => JSON::XS::false,
|
|
border => 'normal',
|
|
'floating_nodes' => $ignore,
|
|
workspace_layout => 'default',
|
|
};
|
|
|
|
# a shallow copy is sufficient, since we only ignore values at the root
|
|
my $tree_copy = { %$tree };
|
|
|
|
for (keys %$expected) {
|
|
my $val = $expected->{$_};
|
|
|
|
# delete unwanted keys, so we can use is_deeply()
|
|
if (ref($val) eq 'SCALAR' and $val == $ignore) {
|
|
delete $tree_copy->{$_};
|
|
delete $expected->{$_};
|
|
}
|
|
}
|
|
|
|
is_deeply($tree_copy, $expected, 'root node OK');
|
|
|
|
my @nodes = @{$tree->{nodes}};
|
|
|
|
ok(@nodes > 0, 'root node has at least one leaf');
|
|
|
|
ok((all { $_->{type} == 1 } @nodes), 'all nodes are of type CT_OUTPUT');
|
|
ok((none { defined($_->{window}) } @nodes), 'no CT_OUTPUT contains a window');
|
|
ok((all { @{$_->{nodes}} > 0 } @nodes), 'all nodes have at least one leaf (workspace)');
|
|
my @workspaces;
|
|
for my $ws (@nodes) {
|
|
my $content = first { $_->{type} == 2 } @{$ws->{nodes}};
|
|
@workspaces = (@workspaces, @{$content->{nodes}});
|
|
}
|
|
|
|
ok((all { $_->{type} == 4 } @workspaces), 'all workspaces are of type CT_WORKSPACE');
|
|
#ok((all { @{$_->{nodes}} == 0 } @workspaces), 'all workspaces are empty yet');
|
|
ok((none { defined($_->{window}) } @workspaces), 'no CT_OUTPUT contains a window');
|
|
|
|
# TODO: get the focused container
|
|
|
|
$i3->command('open')->recv;
|
|
|
|
# TODO: get the focused container, check if it changed.
|
|
# TODO: get the old focused container, check if there is a new child
|
|
|
|
#diag(Dumper(\@workspaces));
|
|
|
|
#diag(Dumper($tree));
|
|
|
|
|
|
done_testing;
|